Spring Core - Aspect-Oriented Programming
|
Aspect-Oriented Programming (AOP) is a programming paradigm that provides a way to modularize cross-cutting concerns in an application. Cross-cutting concerns are aspects of a program that affect multiple modules or components, such as logging, security, transaction management, and performance monitoring. In traditional object-oriented programming (OOP), these concerns are often scattered across many classes, leading to code duplication and reduced maintainability.
Key Concepts of AOP
- Aspect
- An aspect is a modular unit of cross-cutting concern. It encapsulates the behavior that affects multiple components in a single place.
- Example: Logging, security, or transaction management can be implemented as aspects.
- Join Point
- A join point represents a point in the execution of a program where an aspect can be applied.
- Example: Method execution, method calls, field access, or exception handling.
- Advice
- Advice is the action taken by an aspect at a particular join point. It defines what to do at the join point.
- Types of advice:
- @Before: Executes before the join point.
- @After: Executes after the join point (regardless of success or failure).
- @AfterReturning: Executes only if the join point completes successfully.
- @AfterThrowing: Executes if the join point throws an exception.
- @Around: Wraps the join point and can control whether it executes.
- Pointcut
- A pointcut is an expression that matches join points. It defines where (which join points) the advice should be applied.
- Example: A pointcut might match all methods in a specific package or all methods annotated with @Transactional.
- Weaving
- Weaving is the process of linking aspects with the target objects (application code) to create an advised object.
- Types of weaving:
- Compile-Time Weaving: Aspects are woven into the code during compilation.
- Load-Time Weaving: Aspects are woven during class loading.
- Runtime Weaving: Aspects are woven during runtime (common in Spring AOP).
Problems AOP Solves
Separation of Concerns
- AOP allows you to separate cross-cutting concerns (like logging or security) from the core business logic. This improves modularity and maintainability.
Reduces Code Duplication
- Cross-cutting code often appears in multiple places in the application. AOP centralizes this logic into reusable aspects, reducing code repetition.
Enhances Maintainability
- Changes to cross-cutting concerns can be made in one place (the aspect) rather than in multiple locations in the code.
Improves Readability
- Business logic is no longer cluttered with technical concerns, making the code easier to read and understand.
Dynamic Behavior
- AOP allows you to add behavior dynamically to existing code without modifying the code itself, enabling cleaner and more flexible designs.
Simplifies Testing
- Since cross-cutting concerns are decoupled from business logic, testing the core logic becomes easier and more focused.
// without AOP
public class OrderService {
public void placeOrder() {
System.out.println("Logging: Placing order");
// Business logic
System.out.println("Order placed");
}
}
// with AOP
@Aspect
public class LoggingAspect {
@Before("execution(* com.example.OrderService.*(..))")
public void logBefore() {
System.out.println("Logging: Placing order");
}
}
public class OrderService {
public void placeOrder() {
// Business logic
System.out.println("Order placed");
}
}
Example Use Cases for AOP
- Logging
- Automatically log method invocations and exceptions.
- Security
- Enforce security policies such as role-based access control.
- Transaction Management
- Apply transactional behavior across different services.
- Performance Monitoring
- Measure execution times of methods for diagnostics.
- Caching
- Implement caching behavior transparently.
Limitations of AOP
- Complexity:
- AOP can make the program flow harder to understand, as behavior is applied dynamically and is not always evident in the code.
- Overhead:
- AOP adds a layer of processing (e.g., proxies), which can introduce some runtime overhead.
- Limited Scope in Spring AOP:
- Spring AOP only supports method-level join points and is proxy-based. For field-level join points or advanced features, AspectJ (a more powerful AOP framework) is required.
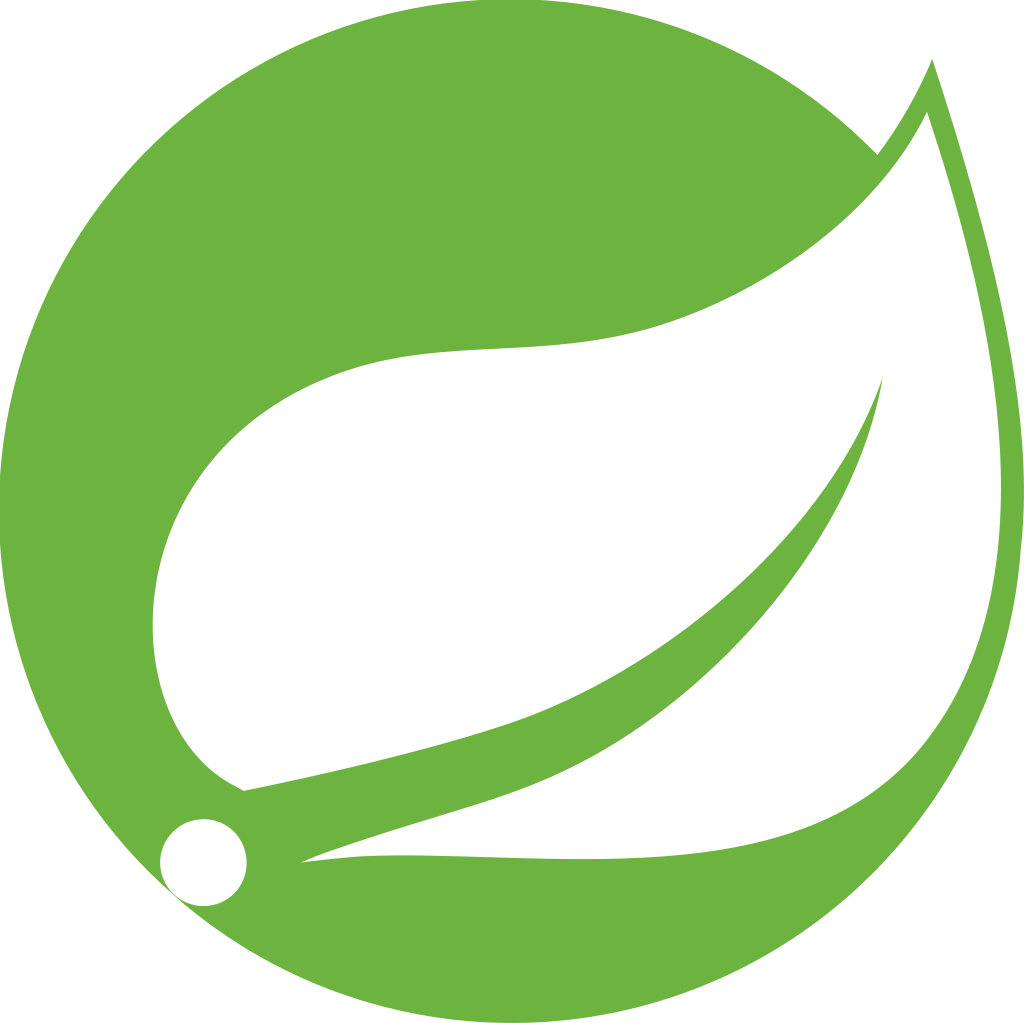