Spring Core - Pointcut Expressions
Pointcut Expressions in Spring AOP define where advice should be applied in your application. They are used to specify join points - specific points in the program execution (e.g., method execution) where cross-cutting behavior (e.g., logging, security, etc.) is applied.
Execution
Matches method execution join points. It’s the most common pointcut type. Same as all the others it's being used inside of an advice annotation like @Before. The annotations in the examples will be omitted. The focus is on the pointcut expressions.
@Advice("execution(modifiers-pattern? return-type-pattern declaring-type-pattern? method-name-pattern(param-pattern) throws-pattern?)")
execution(* com.example.service.*.*(..)) // Methods in the com.example.service package.
execution(public * *(..)) // Any public method
execution(* com.example..*.*(..)) // Methods in any subpackage of com.example
execution(* com.example.service.*.save*(..)) // Methods starting with "save"
execution(* *(..)) // Matches all methods in the application
Within
Matches all methods of a specific class or package.
within(package-name-pattern)
within(com.example.service.*) // Matches all methods in classes inside the com.example.service package
within(com.example.service..*) // Matches methods in the package and its sub-packages
within(com.example.service.OrderService) // Matches all methods in `OrderService` class
@Within
Matches methods in classes annotated with a specific annotation.
@within(annotation-name)
// Matches all methods in classes annotated with @Service.
@within(org.springframework.stereotype.Service)
@Annotation
Matches methods annotated with a specific annotation.
@annotation(annotation-name)
// Matches all methods annotated with @Transactional.
@annotation(org.springframework.transaction.annotation.Transactional)
Target
Matches methods where the target object (proxied object) is of a specific type.
target(class-name-pattern)
// Matches methods in beans where the target object is of type OrderService.
target(com.example.service.OrderService)
@Target
Matches methods in beans where the target object’s class has a specific annotation.
@target(annotation-name)
// Matches methods in objects that have @Service annotation on their class.
@target(org.springframework.stereotype.Service)
Bean
Matches beans by their Spring bean names.
bean(bean-name-pattern)
bean(orderService) // Matches all methods in the Spring bean named orderService.
bean(*Service) // Matches beans with names ending in "Service"
bean(my*Bean) // Matches beans whose names start with "my" and end with "Bean"
Args
Matches methods where the arguments match specified types.
args(argument-type-patterns)
args(java.lang.String, int) // Matches methods with a String and an int as arguments.
args(.., int) // Matches methods with any number of arguments ending with an int
args(String, ..) // Matches methods starting with a String argument
args(..) // Matches methods with any number of arguments
This
Matches methods where the current proxy object is of a specific type.
this(class-name-pattern)
// Matches methods in beans proxied as type OrderService.
this(com.example.service.OrderService)
Combination of Pointcuts
Spring AOP allows you to combine multiple pointcut expressions using logical operators:
- && (AND)
- || (OR)
- ! (NOT)
The following expression matches transactional methods in the com.example.service package.
execution(* com.example.service.*.*(..)) && @annotation(org.springframework.transaction.annotation.Transactional)
Matching Exceptions
Matches methods that declare throwing specific exceptions.
execution(* *(..) throws exception-type)
// Matches methods that declare throwing IOException.
execution(* *(..) throws java.io.IOException)
Best Practices
- Use execution for most method-level matching.
- Use @annotation to target methods with specific annotations (e.g., @Transactional).
- Combine pointcuts for precise targeting (e.g., execution with @annotation).
- Prefer bean pointcuts for bean-specific behavior if method-level matching is insufficient.
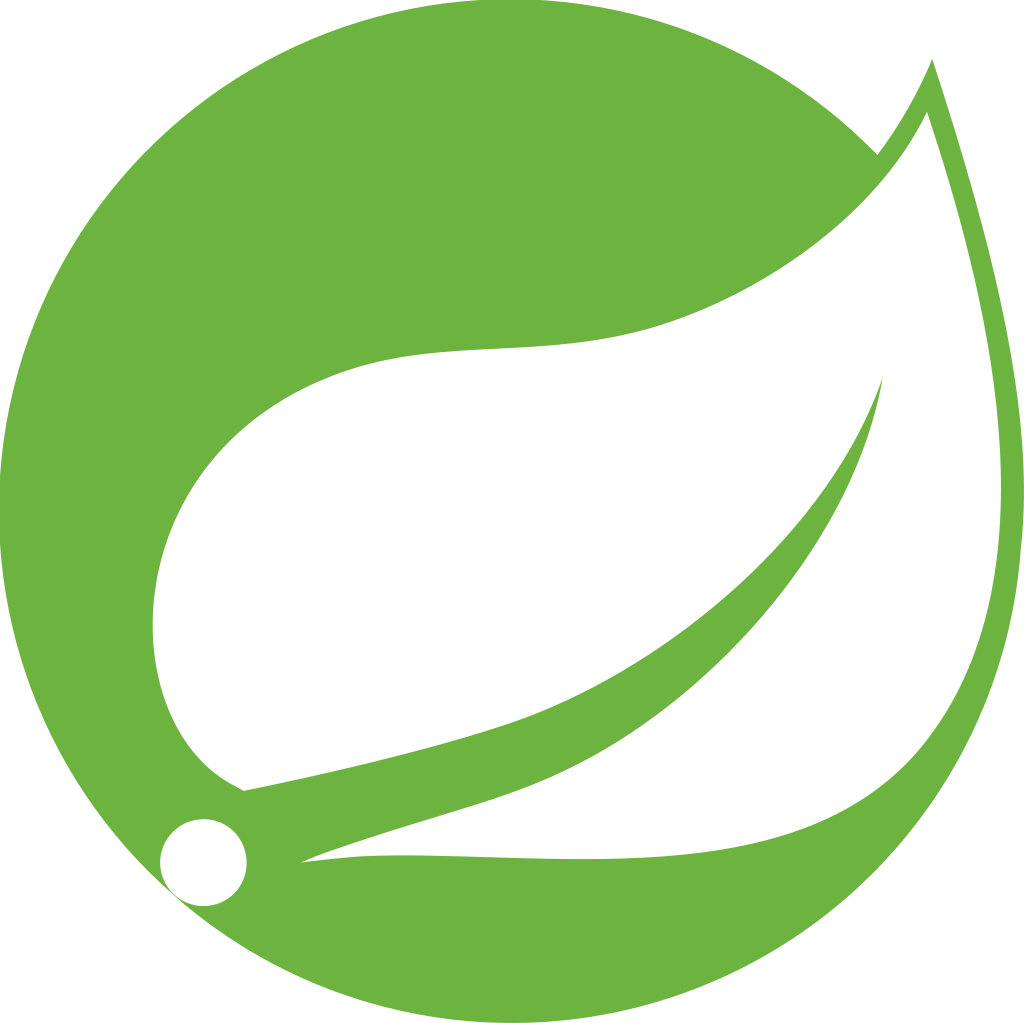