Spring Core - Introduction to Spring Framework
|
This course is an overview of the requirements for the Spring Certified Developer Training. It is as concise as possible, which should allow for faster learning.
Introduction to Spring Framework
The Spring Framework is one of the most widely used frameworks for building Java-based enterprise applications. It provides a comprehensive programming and configuration model for modern Java-based systems, whether on-premise or in the cloud. Designed to address the complexity of enterprise application development, Spring offers a lightweight, modular, and flexible architecture.
Key Features of the Spring Framework
- Lightweight and Modular:
- The core of Spring is lightweight, meaning it does not require heavy resources to get started.
- It is modular, allowing developers to use only the components they need (e.g., Spring MVC, Spring Data, Spring Security).
- Inversion of Control (IoC):
- Central to Spring is the IoC Container, which manages the lifecycle and configuration of objects.
- IoC shifts the responsibility for managing dependencies from the application to the framework, improving testability and flexibility.
- Dependency Injection (DI):
- Spring supports DI, a design pattern that enables you to inject dependencies into an object at runtime, making applications loosely coupled.
- Aspect-Oriented Programming (AOP):
- AOP allows developers to separate cross-cutting concerns (e.g., logging, security) from business logic. Spring’s AOP module simplifies implementing such aspects.
- Data Access and Persistence:
- Spring provides robust integration with databases and ORM frameworks like Hibernate and JPA, making data access seamless.
- It abstracts complex database interactions using the Spring JDBC Template.
- Spring MVC:
- A powerful web framework for building scalable, RESTful, and traditional web applications.
- Based on the Model-View-Controller (MVC) design pattern, it simplifies web application development.
- Spring Boot:
- A project built on Spring that simplifies application development by providing a convention-over-configuration approach.
- With Spring Boot, you can quickly create standalone, production-ready Spring applications with minimal setup.
- Spring Security:
- Provides authentication, authorization, and other security features for securing applications.
- Highly customizable and integrates seamlessly with other Spring components.
- Spring Cloud:
- Helps developers build microservices-based architectures by offering tools for distributed systems, service discovery, load balancing, and more.
- Integration with Third-Party Frameworks:
- Spring supports integration with various third-party libraries, like Apache Kafka, RabbitMQ, and Elasticsearch, for building modern, scalable applications.
Benefits of Using the Spring Framework
- Simplified Application Development:
- By reducing boilerplate code and offering pre-built templates for repetitive tasks.
- Enhanced Testability:
- The decoupled architecture and dependency injection make unit testing easier.
- Community and Ecosystem:
- Spring has a vast and active community, ensuring regular updates, best practices, and a wealth of tutorials.
- Cross-Platform Support:
- Spring applications can run on any platform supporting the Java Virtual Machine (JVM).
- Flexibility:
- Developers can integrate Spring with other technologies and frameworks effortlessly.
Spring Framework Workflow
- Application Configuration:
- Define beans and their dependencies using XML, annotations, or Java-based configuration.
- IoC Container:
- The container instantiates, configures, and manages the lifecycle of beans.
- Dependency Injection:
- Dependencies are injected at runtime, ensuring flexibility.
- Service Layer:
- The business logic layer interacts with data access components and external APIs.
- View Layer:
- For web applications, Spring MVC handles request routing and response rendering.
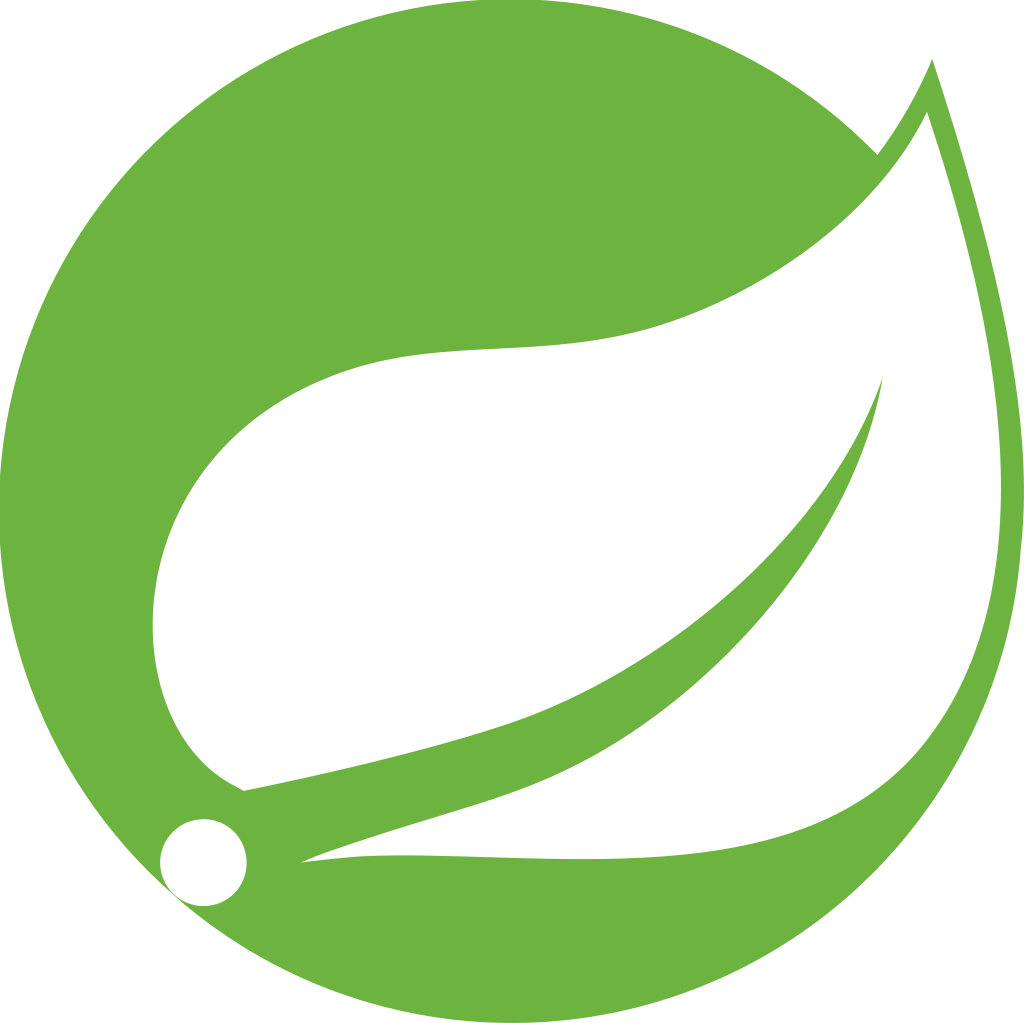